I picked up a Novation Launchkey Mini II controller last year when I was working with those musical floppy drives, and recently I fell down the rabbit hole of Launchpad LED performance videos. That got me thinking: is it possible to control the LEDs on a Launchkey Mini like you can on a Launchpad?
There’s surprisingly little information about this. Novation’s user guide for the Launchkey Mini has no mention of how to control the LEDs. There is some information available in a “programmer’s reference” manual for the Launchkey II (not the “Mini” version), but sadly the LED components don’t function the same way.
It required a bit of reverse engineering and the result isn’t quite as pretty, but I’ve figured out how to do it.
Basic Setup
The Launchkey Mini is a class compliant MIDI device, meaning that it’s possible to talk to it just by sending standardized MIDI messages without needing a custom driver. But before controlling the LEDs, you have to be connected to the right MIDI port and have the device configured for InControl mode.
USB Endpoints
The Launchkey Mini presents itself as a generic USB-MIDI device with two I/O endpoints. The first endpoint is the MIDI port for the control surface data; this is what you connect to for reading the keyboard, drum pads, and potentiometers. The second endpoint is what Novation refers to as the “InControl” port. This is how Ableton sends commands to the device without disrupting the main MIDI stream.
To control the LEDs, you need to connect to this second port. For me this is listed as “Launchkey Mini (Port 2)” in Ableton, and as “MIDIOUT2 (Launchkey Mini) 2” in other software such as Python (mido) and Hairless. If you’re using Ableton, the port must also be set as an output device and enabled for track output in your MIDI preferences.
InControl
The key to this all is the “InControl” mode. Within Ableton, switching to this mode lets you control your currently playing tracks and navigate the interface. More importantly, when the controller is in this mode Ableton is put in control of the LEDs underneath the drum pads.
If you’re controlling the LEDs from Ableton, just press the InControl button on the Launchkey Mini once until it glows red.
If you’re not controlling the LEDs via Ableton, you need to send a message to the Launchkey Mini to switch it to “InControl” mode. The magic message is a “note on” message on channel 1 (0x90) for note 12 (0x0C) at velocity 127 (0x7F). The same message at velocity 0 (0x00) will switch “InControl” mode off. These messages will be mirrored back on the InControl MIDI port to the computer once they are received by the device.
LED Command
The Launchkey Mini has 18 controllable LEDs: two rows of 8 drum pads, and two round buttons.
The LEDs are controlled using a “note on” command on MIDI channel 1 (byte 0x90), where the “note” is the LED to control, and the “velocity” is the color to set.
0x90 {LED #} {Color}
Be aware that a respective “note off” command will not turn off the LED, you need to send an additional “note on” command with the off color.
LED IDs
The LEDs are numbered sequentially from left to right. On the top row, the LEDs start at note C6 (96) and end at note G#6 (104). On the bottom row, the LEDs start at note E7 (112) and end at note C8 (120).
Note: For some bizarre reason, there is debate with MIDI about whether middle C (60) is “C3” or “C4”. I’m going to use C3 = 60 just because that’s how Ableton labels its notes and that’s what the controller was designed around. If you’re using software where C4 = 60, just transpose all of the octaves up by one (e.g. C6 becomes C7). Or use the decimal numbers.
Color Palette
The Launchkey Mini has a limited color palette. For each pad there are only red and green diodes, so you can’t display any colors with a blue component. In other words, no RGB / full color display. That’s a hardware limitation and there is no workaround.
On top of that, you’re limited to the 127 velocities you can specify via the MIDI “note on” message. If that wasn’t bad enough, there are only 16 unique colors available:
Colors increase in red brightness from left to right, and increase in green brightness from top to bottom. For any given color, add 1 to increase the red value and add 16 to increase the green value (up to a max of 3x per component).
For completeness, here is the full palette of available colors. You can see that colors are grouped in fours and then repeated four times, and the entire palette of 64 is duplicated twice.
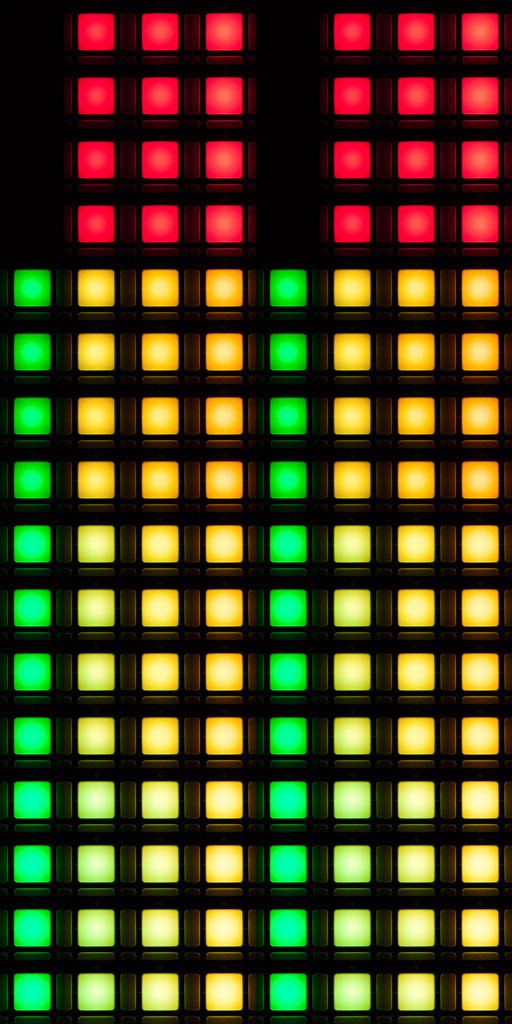
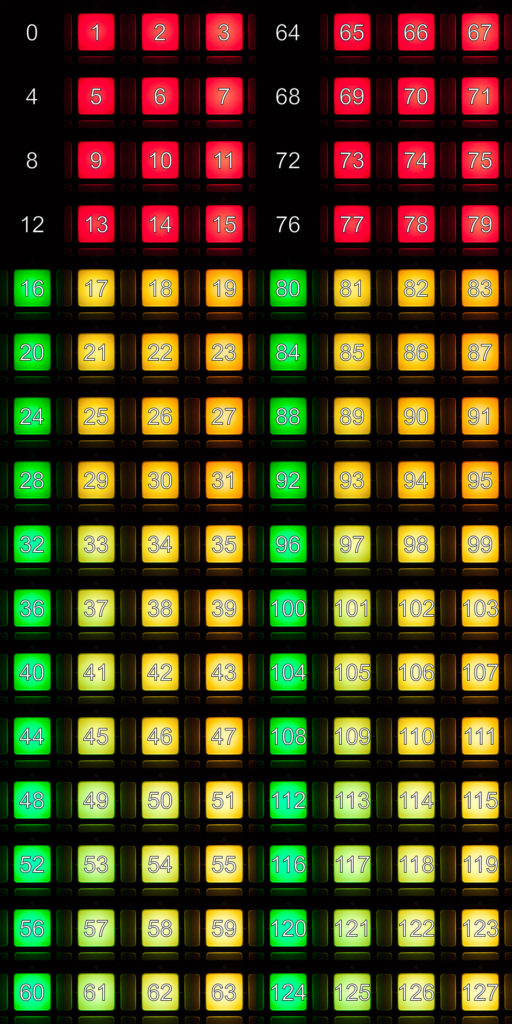
Demo
To demonstrate this new-found power of LED control, I wrote a short sweeping animation in Python. The script generates a random color from the available palette, then progressively writes it to the device’s LEDs from left to right.
The only external dependency here is the mido library, which handles the MIDI communication. Here is the script, in full:
# # Launchkey Mini II Sweep Animation # by David Madison © 2018 # www.partsnotincluded.com # # This is a sweep animation to demonstrate control of the Novation Launchkey Mini # II's RG LED pads. The script will generate a random color from the LED palette # that sweeps from left to right across the available LED pads. # import mido import random from time import sleep row1 = (96, 97, 98, 99, 100, 101, 102, 103, 104) # LED indices, first row row2 = (112, 113, 114, 115, 116, 117, 118, 119, 120) # LED incides, second row leds = row1 + row2 # LED indices, combined def write_led(led_id, color_vel): midi_out.send(mido.Message('note_on', channel=0, note=led_id, velocity=color_vel)) def random_color(): red_or_green = bool(random.randint(0, 1)) # Making sure the number is always >0 for one component return random.randint(int(red_or_green), 3) + \ random.randint(int(not red_or_green), 3) * 16 if __name__ == "__main__": try: midi_out = mido.open_output("MIDIOUT2 (Launchkey Mini) 2") # Launchkey InControl port midi_out.send(mido.Message.from_bytes([0x90, 0x0C, 0x7F])) # Switch to "InControl" mode while True: color = random_color() for index, led in enumerate(row1): # Set current LED color write_led(row1[index], color) write_led(row2[index], color) # Turn off last set LEDs write_led(row1[index - 1], 0) write_led(row2[index - 1], 0) sleep(0.1) except KeyboardInterrupt: pass for led in leds: write_led(led, 0) # Turn off all LEDs midi_out.send(mido.Message.from_bytes([0x90, 0x0C, 0x00])) # Disable "InControl" mode midi_out.close()
Conclusion
To recap: in order to control the LEDs on your Novation Launchkey Mini II, you need to connect to the second MIDI output port and set InControl mode to “on” (either via Ableton or using the special message 0x90 0x0C 0x7F
). Then to change colors you use another “note on” message where the LED position is your “note” and the color of choice is your “velocity”. That’s it!
If you found this post helpful, like, follow, and subscribe check out some of my other projects.
Getting InControl
When I was originally fiddling around with this, I figured out I could get the LEDs to respond to messages from Ableton so long as the “InControl” mode was enabled, and yet I couldn’t switch the device into “InControl” mode without having Ableton open. There was no information about switching modes outside of Ableton in the user manual, and after contacting Novation support they were unable to help me either. So I put on my reverse engineering cap and got to work.
USB-MIDI
The Launchkey Mini is a class-compliant USB device, which means it must behave by the publicly available (and well-documented) USB MIDI standard (pages 16-17 are particularly helpful). I installed a copy of Wireshark (2.6.2) which came with USBPcap for analyzing USB packets on Windows. I then plugged in the Launchkey Mini II, started up Ableton, and watched as the messages flew by.
After a flurry of messages, the device sends a message back:
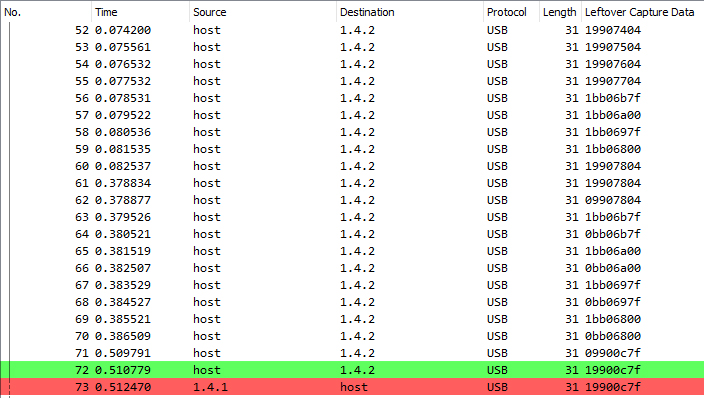
This message mirrors what was sent by Ableton, indicating that it’s an acknowledgement of something important.
Unpacking
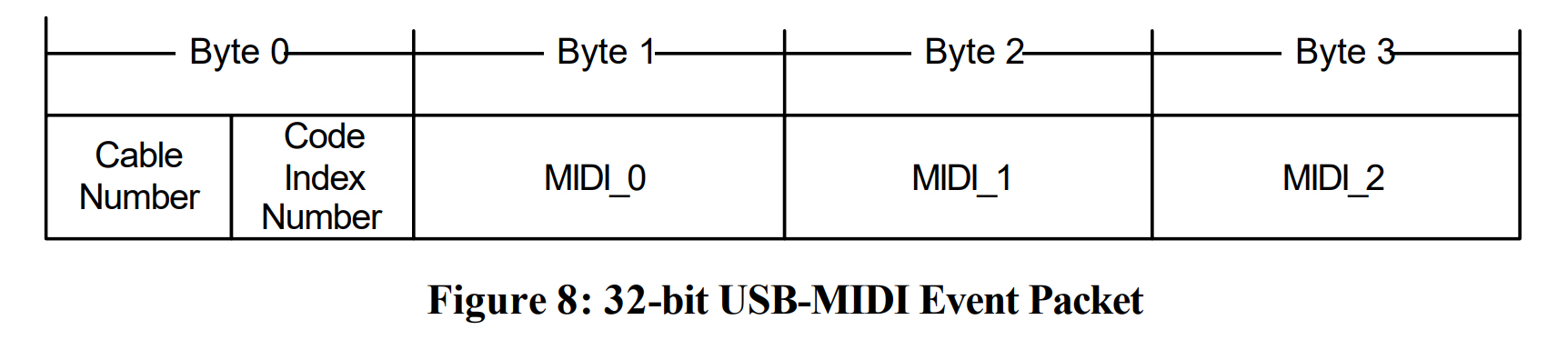
Ignoring the extra USB header information, the message is composed of four bytes, which matches a standard USB-MIDI event packet. The four-byte packet sent from Ableton is:
0x19 0x90 0x0C 0x7F
The first byte is USB specific. The first nybble, 1
, indicates that it’s a message intended for the second (indexed at 0) “virtual cable”. In other words, it’s meant for the second virtual MIDI device on the microcontroller – the InControl MIDI port. The second nybble, 9
, shows that it’s a “note-on” message.
The following three bytes are your standard serialized MIDI message. 0x90
means it’s a “note-on” message on channel 1, 0x0C
means it’s for note 12, and 0x7F
means it has a velocity of 127. I threw these values into a Python script and the InControl light came on immediately.
22 Comments
Chris · April 27, 2019 at 10:47 pm
Hi Dave,
Thanks for this great article. I could get my Launhkey mini mk2 to light up to a midi track in Ableton live 10 lite, but somehow the lights just flash at their own rate and not to the pattern in the midi track.
For example, I just made a midi track with one beat on each key E7, F7, F#7, G7 but the four pads on the bottom row just flash constantly instead of pulsing to the tempo as I expected. Adjusting the velocities in Ableton produces different colours but don’t stop the flashing. All the flashing stops once I create and delete a new midi track.
I set the midi track to channel 1, but when I set it to channel 3 it’s unresponsive for nearly a minute then suddenly all the pads flash with some random flickering.
Hope you may have some idea how I can fix this. Thanks.
Dave · April 28, 2019 at 4:00 am
Hi Chris,
Try some of the higher velocity numbers for the colors. I know that 127 (yellow) to 8 (off) works in my version of Ableton.
I pulled out the keyboard for more testing and it looks like you’re right – I can get the LEDs to flash in Ableton just by tweaking the velocity numbers. Since I didn’t experience that when writing the MIDI data myself, it must be triggered by the corresponding “note off” messages. I’ll have to come back to this when I have the time and do some more digging.
Timothy · May 11, 2019 at 9:27 am
Hi Dave
.. I recently connected my Novation Launchkey 25 to my laptop but it has refused to work… When I connect it, all the Led lights come on and they stay on and the number displayed on the screen is ‘8.8.8.’.. But the novation does not work at all and the lights do not go off at all… Do you have any idea of what could be wrong and what I can do to fix it?
Dave · May 11, 2019 at 5:33 pm
This article is about the Launchkey Mini, not the Launchkey 25. I have no experience with the Launchkey 25. I recommend you contact Novation support.
Niels · August 30, 2019 at 6:31 am
Hi Dave, did you ever try to flash/pulse the LEDs against a MIDI clock signal? I tried to use the MIDI messages as mentioned in the programmer’s reference for the LaunchKey Mk2 series (which indeed does not cover the our Mini Mk2) but without success. The majority of the MIDI messages in that manual actually work nicely (i.e. enabling extended mode, turning on LEDs).
I used this document as a reference: https://d2xhy469pqj8rc.cloudfront.net/sites/default/files/novation/downloads/10535/launchkey-mk2-programmers-reference-guide.pdf
Dave · August 30, 2019 at 10:21 am
Unfortunately I never got that far in my reverse engineering. I was just trying to figure out how to control the LEDs manually with a script, not link them to a MIDI source of any sort.
You could certainly create a work-around with a small program reading from the MIDI stream – on a clock pulse, send the prepacked LED on/off command and toggle a flag to send the inverse on the next pulse. Though that will require passing the entire MIDI stream through that program, which is obviously less than ideal.
That programming guide is useful, but as you discovered it’s not accurate for the Launchkey Mini (though there is some overlap).
Tyler · March 15, 2020 at 3:45 pm
Hey I cannot make sense how to apply this information to Ableton 10 and my Launchkey mini mk2
Could you make a step by step tutorial video on how to apply this information?
Thanks
Bill Mill · July 7, 2020 at 10:17 am
Thank you for this information!
Just for any other users who come across this: this unfortunately doesn’t work on the MK3; I plan to investigate but who knows.
Bill Mill · July 7, 2020 at 4:21 pm
Update: figured it out https://gist.github.com/llimllib/13a391bc52aea9af3c8e81a315647240
Dave · July 7, 2020 at 4:32 pm
Nicely done! Thanks for sharing Bill.
Yared · July 17, 2020 at 6:36 pm
Is it possible to launch the RGB without any DAW?
Just plug in to the computer and start a program that launches the RGB?
Dave · July 17, 2020 at 6:39 pm
Absolutely, you just need a program that “speaks” MIDI. See the Python script above.
Although again the MkII does not have full color (RGB) support: just red and green with a total of 16 colors.
Alex · August 7, 2020 at 5:48 am
Hello! Is it possible to activate InControl by using Arduino with USB Host Shield?
Dave · August 7, 2020 at 5:58 am
Absolutely, just send the MIDI message on the right interface. You may need to talk directly to the host shield via SPI if the library you’re using doesn’t support multiple MIDI interfaces, however.
Alex · August 7, 2020 at 7:09 am
Damn, sounds like a sorcery to me. I’ve tried to just to send 4-byte message to controller, but nothing happend. Guess, I need to send it exactly to second MIDI interface. But don’t have any idea how to do it.
Anyway, big thanks for your answer
Paul · May 12, 2021 at 3:10 pm
Hi Dave, thanks for the Python code.
To run it on the MK3, I only had to change line 32 & 33 to:
32 midi_out = mido.open_output(‘MIDIOUT2 (Launchkey Mini MK3 MI 2’) # Launchkey InControl port
33 midi_out.send(mido.Message.from_bytes([0x9F, 0x0C, 0x7F])) # Switch to “InControl” mode
The magic message is a “note on” message on channel 16 (0x9F), see this page: https://github.com/giezu/LaunchkeyMiniMK3
Dave · May 13, 2021 at 8:09 am
That’s great info, thanks Paul!
ismaele · August 15, 2022 at 2:51 pm
Hi Dave thank you for the script and for the informations, unfortunately when i start my program it raise this error:
Traceback (most recent call last):
File “/Users/——-/PycharmProjects/midi/prova.py”, line 32, in
midi_out.send(mido.Message.from_bytes([0x90, 0x0C, 0x7F])) # Switch to “InControl” mode
AttributeError: ‘NoneType’ object has no attribute ‘send’
idk if you will reply me but i thank you anyway!
Dave · August 18, 2022 at 1:10 pm
Hi Ismaele. That’s a sign that it couldn’t open the MIDI port so there’s no device to write to. Make sure the device is connected and no other software is trying to use the MIDI port.
Julian · October 7, 2023 at 12:15 am
Thanks for this. I’ve got a LaunchKey Mini Mk I, and my script has control over the LEDs now.
Is my understanding correct?:
When InControl is off (default), the LaunchPads buttons act like keys on Channel 9. They flash red when depressed, and NoteOn/NoteOff is sent over MIDI.
When the InControl button is (manually) held down like a shift key, the LaunchPad buttons select what channel the keyboard uses. The current selected channel is lit green. This is not transmitted over MIDI.
When the InControl button is physically pressed quickly (or virtually held down via MIDI), it changes to InControl mode. Then (and only then) you can affect the launchpad colours, but nothing is sent over MIDI when they are actually pressed.
So, I can’t light up a Launchpad button in Amber, and then detect when it is pressed.
Dave · October 9, 2023 at 9:36 am
When the InControl mode is enabled MIDI data is still sent, it just changes interfaces. The device has two virtual MIDI ports: one which acts as the standard MIDI I/O for the keyboard and one which is a meta interface for the DAW.
When the InControl mode is disabled all controls inputs (keys, pads, rotary knobs) are sent on interface 1.
When the InControl mode is enabled (via the special packet on interface 2), the pads and rotary knobs switch from interface 1 to interface 2 and report on channel 1.
So yes – you can set the color of the button and detect when it’s pressed, you just need to read from the second interface.
Julian · October 9, 2023 at 8:47 pm
Ah, understood. That is working perfectly for me. Thank you so much for helping a random on the Internet. You are my favourite person in the world for today.